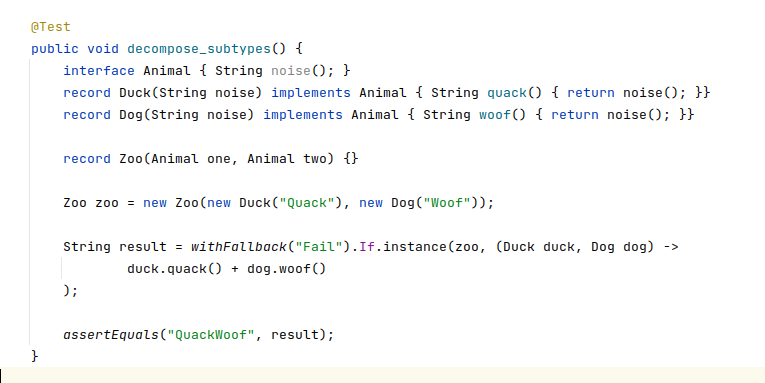
Java 16 brings Pattern Matching for instanceof. It’s a feature with exciting possibilities, though quite limited in its initial incarnation. Basic Pattern Matching We can now do things like Object o = "hello"; if (o instanceof String s) { System.out.println(s.toUpperCase()); }Object o = "hello"; if (o instanceof String s) { System.out.println(s.toUpperCase()); } Note the variable… Read more »